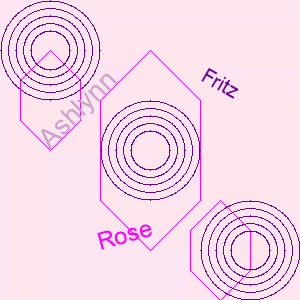
Ashlynn's Unique Drawing Example
Ashlynn's Unique Drawing Example
<?php
include('includes/header.php');
// create the image
$image = imagecreatetruecolor(300, 300);
// background color
$background = imagecolorallocate($image, 255, 230, 240);
imagefilledrectangle($image, 0, 0, 300, 300, $background);
// colors
$darkPurple = imagecolorallocate($image, 102, 0, 153);
$lightPink = imagecolorallocate($image, 255, 182, 193);
$magenta = imagecolorallocate($image, 255, 0, 255);
$lilac = imagecolorallocate($image, 200, 162, 200);
// draw hexagons
$hexagons = [
[150, 50, 200, 100, 200, 200, 150, 250, 100, 200, 100, 100], // center
[50, 50, 80, 80, 80, 120, 50, 150, 20, 120, 20, 80], // top left
[220, 200, 250, 230, 250, 270, 220, 300, 190, 270, 190, 230] // bottom right
];
foreach ($hexagons as $hexagon) {
imagepolygon($image, $hexagon, 6, $magenta);
}
// draw concentric circles
$circlePositions = [[150, 150], [50, 50], [250, 250]];
foreach ($circlePositions as $pos) {
for ($i = 40; $i <= 100; $i += 15) {
imagearc($image, $pos[0], $pos[1], $i, $i, 0, 360, $darkPurple);
}
}
// add rotated text
$font = __DIR__ . '/fonts/arial1.ttf';
$text = "Ashlynn";
imagettftext($image, 20, 45, 50, 150, $lilac, $font, $text); // Rotated 45 degrees
imagettftext($image, 15, -30, 200, 80, $darkPurple, $font, "Fritz"); // Rotated -30 degrees
imagettftext($image, 18, 15, 100, 250, $magenta, $font, "Rose"); // Rotated 15 degrees
// save the image as a file
$filePath = 'generated_image.gif';
imagegif($image, $filePath);
imagedestroy($image);
// display image
echo '<img src="' . $filePath . '" alt="Creative Art">';
echo '<p>Ashlynn\'s Unique Drawing Example</p>';
include('includes/footer.php');
?>